Quick Start Guide for the VCL Edition (Delphi & C++Builder)
Last modified:
1st way: Placing the Active Query Builder control on a form.
Follow the steps below to place a component on a form using Delphi IDE.
- Place the TacQueryBuilder component on the form.
-
Place required metadata and syntax provider components on the form (read more about syntax and metadata providers). Define database connection for the metadata provider.
-
Link the components above to the TacQueryBuilder by setting MetadataProvider and SyntaxProvider properties.
- Place the TacSQLBuilderPlainText component on the form to get SQL code generated by the Query Builder with formatting. Link it to the TacQueryBuilder component by setting its QueryBuilder property.
-
Add the TMemo or any other text editing component (for example, TSynEdit) to a form.
-
Now you should establish a connection between the TacSQLBuilderPlainText and the TMemo components.
Enter the following code to OnExit event of TMemo component:acQueryBuilder1.SQL := Memo1.Text;
Enter the following code to OnSQLUpdated event of TacSQLBuilder component:
Memo1.Text := acSQLBuilderPlainText1.SQL;
- Execute the following code to load metadata and activate Active Query Builder:
acQueryBuilder1.RefreshMetadata; // syncronous loading; may take a long time in case of large database schema
or
acQueryBuilder1.RefreshMetadataAsync; // asyncronous loading; allows to work with the component almost instantly. // database schema tree will be filled after completion of the metadata loading process.
-
That's all! Now you can run your application.
Don't forget to activate your database connection component.
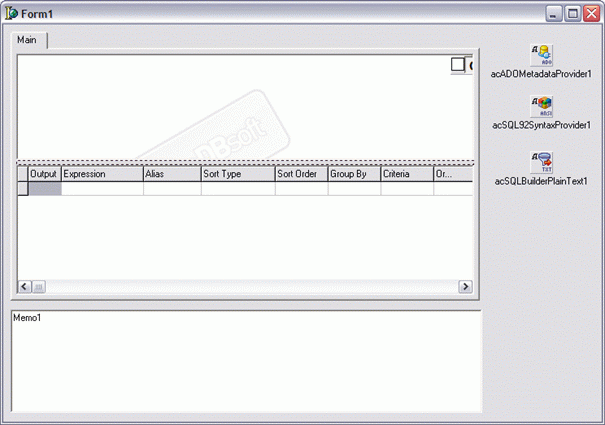
2nd way: Creating a component programmatically.
The following sample illustrates the programmatic creation of needed objects to work with the component.
unit uActiveQueryBuilder; uses // ... acAST, acQBBase; interface type TForm1 = class(TForm) // ... private adoConnection: TADOConnection; acQueryBuilder: TacQueryBuilder; // specify the needed type of metadata provider according to your database connection acMetadataProvider: TacADOMetadataProvider; // specify the needed type of syntax provider for your database server acSyntaxProvider: TacMSAccessSyntaxProvider; acPlainTextSQLBuilder: TacSQLBuilderPlainText; // ... end; implementation procedure TForm1.FormCreate(Sender: TObject); begin acQueryBuilder := TacQueryBuilder.Create(Self); acMetadataProvider := TacADOMetadataProvider.Create(Self); acSyntaxProvder := TacMSAccessSyntaxProvider(Self); adoConnection := TADOConnection.Create(Self); adoConnection.ConnectionString := 'Microsoft.ACE.OLEDB.12.0;Data Source=C:\myFolder\myAccessFile.accdb;Persist Security Info=False;'; acMetadataProvider.Connection := adoConnection; acQueryBuilder.MetadataProvider := acMetadataProvider; acQueryBuilder.SyntaxProvider := acSyntaxProvider; acPlainTextSQLBuilder := TacSQLBuilderPlainText.Create(Self); acPlainTextSQLBuilder.QueryBuilder := acQueryBuilder; // get the needed metadata to fill the Database Schema Tree: acQueryBuilder.RefreshMetadata; // specify the initial SQL if needed: acQueryBuilder.SQL := 'SELECT * FROM Table1'; // display the formatted SQL query to the user: ShowMessage(acPlainTextSQLBuilder.SQL); end;